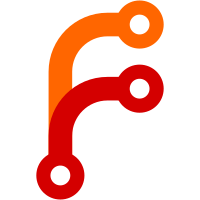
I'm taking a page from Dolphin's book, and including copies of each dependency's source code. This combines the ease of use of including pre-built libraries instead of needing to navigate a package manager - as is (or was) the case for MSVC - with the portability of using packages. Granted, this method's more of a jack of all trades, master of none, since it's *less* user-friendly than prebuilt packages (compilation times), and you don't get the per-distro compatibility fixes you'd get from a package manager. You can still use system libs if you want. In fact, it's still the default behaviour: compiling the libs manually is just a fallback. I'll add an option to force-enable this soon, however, since it's a nicer way to produce static MSYS2 builds than the hackish nightmare that I was using before. Not to mention, having my own copy of the sources means I can provide my own fixes and tweaks your package manager may not. For example, I can combine MSYS2's FreeType subpixel rendering with vcpkg's fix for SDL2 exporting its symbols in static builds.
38 lines
909 B
C++
38 lines
909 B
C++
//
|
|
// "$Id$"
|
|
//
|
|
// Hello, World! program for the Fast Light Tool Kit (FLTK).
|
|
//
|
|
// Copyright 1998-2010 by Bill Spitzak and others.
|
|
//
|
|
// This library is free software. Distribution and use rights are outlined in
|
|
// the file "COPYING" which should have been included with this file. If this
|
|
// file is missing or damaged, see the license at:
|
|
//
|
|
// http://www.fltk.org/COPYING.php
|
|
//
|
|
// Please report all bugs and problems on the following page:
|
|
//
|
|
// http://www.fltk.org/str.php
|
|
//
|
|
|
|
#include <FL/Fl.H>
|
|
#include <FL/Fl_Window.H>
|
|
#include <FL/Fl_Box.H>
|
|
|
|
int main(int argc, char **argv) {
|
|
Fl_Window *window = new Fl_Window(340,180);
|
|
Fl_Box *box = new Fl_Box(20,40,300,100,"Hello, World!");
|
|
box->box(FL_UP_BOX);
|
|
box->labelfont(FL_BOLD+FL_ITALIC);
|
|
box->labelsize(36);
|
|
box->labeltype(FL_SHADOW_LABEL);
|
|
window->end();
|
|
window->show(argc, argv);
|
|
return Fl::run();
|
|
}
|
|
|
|
//
|
|
// End of "$Id$".
|
|
//
|
|
|