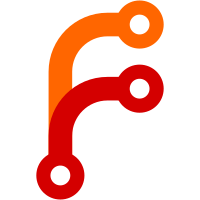
This is currently hardcoded to 640x480 English builds. What I've done is temporarily gutted the FreeType2 font renderer, and instead introduced a system where the font is read from a pre-rendered font atlas. This allows me to get 100% accurate font rendering to Windows XP... because I literally created these atlases with Windows XP. So not only does this eliminate the issue of FreeType producing misshapen glyphs, but it also works around the copyright issue regarding bundling the Courier New and MS Gothic fonts with CSE2. You see, font files can be copyrighted like any old software, however typefaces have a lot less protection - they're basically fair-game. IANAL, of course - look it up yourself. I don't really want to throw away the FreeType font system since I spent a ton of time working on it, it allows you to use other font files, and I'll probably wind up needing it for CSE2EX. I guess I'll just have to find some way to either have the two systems coexist, or make it so one or the other can be selected at compile-time.
47 lines
1.1 KiB
C++
47 lines
1.1 KiB
C++
#include "Bitmap.h"
|
|
|
|
#include <stddef.h>
|
|
#include <stdlib.h>
|
|
|
|
#define STB_IMAGE_IMPLEMENTATION
|
|
#define STB_IMAGE_STATIC
|
|
#define STBI_ONLY_BMP
|
|
#define STBI_ONLY_PNG
|
|
#define STBI_NO_LINEAR
|
|
#define STBI_NO_STDIO
|
|
#include "../external/stb_image.h"
|
|
|
|
#include "File.h"
|
|
|
|
unsigned char* DecodeBitmap(const unsigned char *in_buffer, size_t in_buffer_size, size_t *width, size_t *height, unsigned int bytes_per_pixel)
|
|
{
|
|
int int_width, int_height;
|
|
unsigned char *image_buffer = stbi_load_from_memory(in_buffer, in_buffer_size, &int_width, &int_height, NULL, bytes_per_pixel);
|
|
|
|
*width = int_width;
|
|
*height = int_height;
|
|
|
|
return image_buffer;
|
|
}
|
|
|
|
unsigned char* DecodeBitmapFromFile(const char *path, size_t *width, size_t *height, unsigned int bytes_per_pixel)
|
|
{
|
|
size_t file_size;
|
|
unsigned char *file_buffer = LoadFileToMemory(path, &file_size);
|
|
|
|
if (file_buffer != NULL)
|
|
{
|
|
unsigned char *image_buffer = DecodeBitmap(file_buffer, file_size, width, height, bytes_per_pixel);
|
|
|
|
free(file_buffer);
|
|
|
|
return image_buffer;
|
|
}
|
|
|
|
return NULL;
|
|
}
|
|
|
|
void FreeBitmap(unsigned char *buffer)
|
|
{
|
|
stbi_image_free(buffer);
|
|
}
|